int dup (int filedes);
int dup2 (int filedes , int filedes2);
•기능: 사용 중인 파일 디스크립터를 복사
•리턴 값: 성공하면 할당 받은 파일 디스크립터 번호, 실패하면 -1
–dup( ) 함수는 할당 가능한 가장 작은 번호를 리턴한다.
–dup2( ) 함수는 filedes2를 리턴한다.
•파일 테이블의 해당 항목의 참조 계수를 1 증가 시킨다.
파일 디스크립터 항목의 플래그 (FD_CLOEXEC)는 초기값 (0) 으로 설정된다
•dup2( ) 의 기능
–dup2(fd, STDIN_FILENO) : fd를 표준 입력으로 redirection
–dup2(fd, STDOUT_FILENO) : fd를 표준 출력으로 redirection
–dup2(fd, STDERR_FILENO) : fd를 표준 에러로 redirection
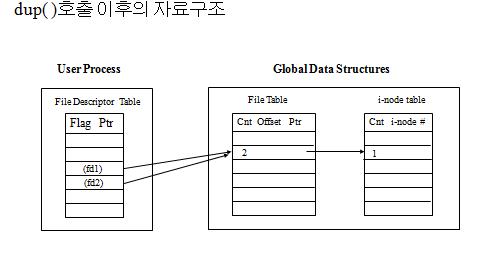
dup()
#include <fcntl.h>
#include <unistd.h>
#include <string.h>
#include <unistd.h>
#include <string.h>
int main()
{
char *hello = "test.txt";
{
char *hello = "test.txt";
int fd1, fd2;
int cnt;
char buffer[30];
int cnt;
char buffer[30];
if((fd1 = open(hello, O_RDONLY)) < 0 )
{
perror("open()");
exit(-1);
}
{
perror("open()");
exit(-1);
}
cnt = read(fd1, buffer, 8);
buffer[cnt] = '\0';
buffer[cnt] = '\0';
printf("printf : %s\n", buffer);
lseek(fd1, 1, SEEK_CUR);
cnt = read(fd1, buffer, 5);
buffer[cnt] = '\0';
lseek(fd1, 1, SEEK_CUR);
cnt = read(fd1, buffer, 5);
buffer[cnt] = '\0';
printf("printf : %s\n", buffer);
return 0;
}
}
[test.txt의 내용]
Hello, UNIX! How are you?
[수행 결과]
fd1's printf : Hello, Unix!
fd2's printf : How are you?
/////////////////////////////////////////////////////////////////////////////////////////
Dup2()
#include <fcntl.h>
#include <string.h>
#include <stdio.h>
#include <unistd.h>
int main()
{
char *hello = "test.txt";
char *hello = "test.txt";
int fd1,fd2;
char buffer[20];
int cnt;
char buffer[20];
int cnt;
if((fd1 = open(hello, O_RDWR)) < 0)
{
perror("open() error");
exit(-1);
perror("open() error");
exit(-1);
}
fd2 = dup2(STDOUT_FILENO,fd1 );
//cnt = read(fd1, buffer ,6 );
write(fd1, "adasdasdqwe", 11 );
// buffer[cnt] = '\0';
// printf("first %s \n", buffer);
fd2 = dup2(STDOUT_FILENO,fd1 );
//cnt = read(fd1, buffer ,6 );
write(fd1, "adasdasdqwe", 11 );
// buffer[cnt] = '\0';
// printf("first %s \n", buffer);
// lseek(fd2, 1, SEEK_CUR);
//cnt = read(fd2, buffer, 4);
// buffer[cnt] = '\0';
// printf("first %s \n", buffer);
//cnt = read(fd2, buffer, 4);
// buffer[cnt] = '\0';
// printf("first %s \n", buffer);
return 0;
}
** 알아서 돌려보시라...*** 해석 잘하면서..
///////////////////////////////////////////////////////////////////////
dup2()
#include <fcntl.h>
main()
{
char *fname = "test.txt";
int fd;
if((fd = creat(fname, 0666)) < 0) {
perror("creat( )");
exit(-1);
}
printf("First printf is on the screen.\n");
dup2(fd,1);
printf("Second printf is in this file.\n");
}
[수행 결과]
% a.out
First printf is on the screen.
%cat test.txt
Second printf is in this file'컴퓨터 > 언어,프로그래밍' 카테고리의 다른 글
hole있는 파일 생성(creat, write, lseek) (0) | 2009.05.27 |
---|---|
운영체제(유닉스)에서 open()과 read()함수의 소스코드 (0) | 2009.05.27 |
Dup2 를 이용한 Redirection 과 원상복귀 (0) | 2009.05.27 |
Socket inheritance with fork/dup2/exec (0) | 2009.05.27 |
간단한 pipe()에 대한 소스 (0) | 2009.05.27 |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |